Create the Web API project
Once the controller had been created, now it's time to add Swagger into our project, for that we need to add a NuGet Package – Swashbuckle.
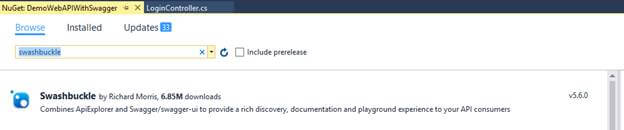
Once you have installed Swashbuckle to your project you can find a SwaggerConfig file in App_Start.

By default Swagger does not show XML Comments, there is an option to display them with Swagger UI, but for that, we need to make sure, when the project is built all the XML comments get saved into the XML file. For that, first, go to project properties. In the Build Section check > XML documentation file, you will then get a path. Mine is bin\DemoWebAPIWithSwagger.XML
Save it with Ctrl + S,
Now, go to SwaggerConfig file shown above, here search for c.IncludeXmlComments(GetXmlCommentsPath()) and uncomment it. Now
you can generate the method using intellisense or you can write a method on the end of the config file.
- private static string GetXmlCommentsPath()
- {
- return System.AppDomain.CurrentDomain.BaseDirectory + @"\bin\DemoWebAPIWithSwagger.XML";
- }
NoteChange the type of method to string.
We are all done now... Let’s run our project. AFter page opens Add /Swagger in url = > http://localhost:5543/swagger
Now you will get a page like this
ou can find our Swagger UI Here,
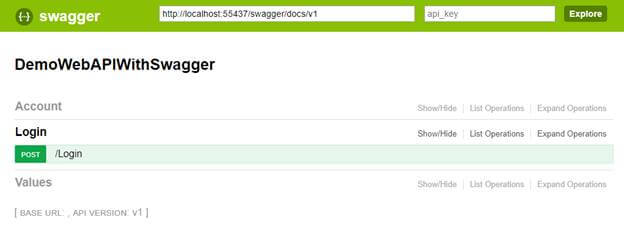
ASP.Net Web API 2 CORS Support:
Enabling Cross Origin Resource Sharing in ASP.Net Web API 2 is simple, once it is enabled any client sending AJAX requests from webpage (on a different domain) to our API will be accepted.
By default CORS assembly doesn’t exist within ASP.NET Web API 2 assemblies so we need install it from NuGet, so open your NuGet package console, and type the following Install-Package Microsoft.AspNet.WebApi.Cors -Version 5.0.0 once the package is installed open “WebApiConfig” class and add the following line of code inside the register method config.EnableCors(); by doing this we didn’t enable CORS yet, there are different levels to enable CORS on ASP.Net Web API, levels are:
1. On controller level
You can now add attribute named EnableCors on the entire controller so by default every action on this controller will support CORS as well, to do this open file “CoursesController” and add the highlighted line of code below:
The EnableCors attribute accept 3 parameters, in the first one you can specify the origin of the domain where requests coming from, so if you want to allow only domain www.example.com to send requests for your API; then you specify this explicitly in this parameter, most of the cases you need to allow * which means all requests are accepted from any origin. In the second parameter you can specify if you need a certain header to be included in each request, so you can force consumers to send this header along with each request, in our case we will use * as well. The third parameter is used to specify which HTTP verbs this controller accepts, you can put * as well, but in our case we want to allow only GET and POST verbs to be called from requests coming from different origin.
In case you want to exclude a certain action in the controller from CORS support you can add the attribute DisableCors to this action, let we assume we want to disable CORS support on method GetCourse so the code to disable CORS support on this action will be as the below:
2. On action level
Enabling CORS on certain actions is fairly simple, all you want to do is adding the attribute EnableCors on this action, as well EnableCors accepts the same 3 parameters we discussed earlier. Enabling it on action level will be as the below code:
3. On the entire API
In some situations where you have many controllers and you want to allow CORS on your entire API, it is not convenient to visit each controller and add EanbleCors attribute to it, in this situation we can allow it on the entire API inside the Register method in Class WebApiConfig, so open your WebApiConfig class and add the code below:
By adding this we enabled CORS support for every controller we have in our API, still you can disable CORS support on selected actions by adding DisableCors attribute on action level.
No comments:
Post a Comment