Background
Connectivity between applications is a very
important aspect from a business applications perspective. Nowadays there are a
lot of mobile applications and single page applications are being created
and such applications needs a strong service end that can provide these
applications with the data and CRUD operations on the data.
SOAP and ASP.NET Web services
Traditionally, Web Services provided a great way
of creating connected web applications. SOAP and XML created an excellent
solution for creating connected web applications. SOAP is a standard XML based
protocol that communicated over HTTP. We can think of SOAP as message format
for sending messaged between applications using XML. It is independent of
technology, platform and is extensible too. ASP.NET Web services provided an
excellent way of creating SOAP based web services.
Problems with SOAP
The SOAP offered an excellent way of transferring
the data between the applications. but the problem with SOAP was that along
with data a lot of other meta data also needs to get transferred with each
request and response. This extra information is needed to find out the
capabilities of the service and other meta data related to the data that is
being transferred coming from the server. This makes the payload heavy even for
small data. Also, Web services needed the clients to create the proxy on their
end. These proxies will do the marshaling and un-marshaling of SOAP WSDL and
make the communication between the application and the web service possible.
The problem with this proxy is that if the service is updated and the proxy on
the client is not then the application might behave
incorrectly.
Welcome to REST
REST stands for Representational State Transfer.
This is a protocol for exchanging data over a distributed environment. The main
idea behind REST is that we should treat our distributed services as a resource
and we should be able to use simple HTTP protocols to perform various
operations on that resource.
When we talk about the Database as a resource we
usually talk in terms of CRUD operations. i.e. Create, Retrieve, Update and
Delete. Now the philosophy of REST is that for a remote resource all these operations
should be possible and they should be possible using simple HTTP protocols.
Now the basic CRUD operations are mapped to the
HTTP protocols in the following manner:
§
GET: This maps to the R(Retrieve) part of the CRUD
operation. This will be used to retrieve the required data (representation of
data) from the remote resource.
§
PUT: This maps to the U(Update) part of the CRUD
operation. This protocol will update the current representation of the data on
the remote server.
§
POST: This maps to the C(Create) part of the CRUD
operation. This will create a new entry for the current data that is being sent
to the server.
§
DELETE: This maps to the D(Delete) part of the CRUD
operation. This will delete the specified data from the remote server.
so if we take an hypothetical example of a remote
resource that contain a database of list of books. The list of books can be
retrieved using a URL like:
www.testwebsite.com/books
To retrieve any specific book, lets say we have
some ID that we can used to retrieve the book, the possible URL might look
like:
www.testwebsite.com/books/1
Since these are GET requests, data can only be
retrieved from the server. To perform other operations, if we use the similar
URI structure with PUT, POST or DELETE operation, we should be able to
create, update and delete the resource form the server. We will see how this
can be done in implementation part.
Note: A lot
more complicated queries can be performed using these URL structures. we will
not be discussing the complete set of query operations that can be performed
using various URL patterns.
Now the if we compare the REST API wit SOAP, we
can see the benefits of REST. Firstly only the data will be traveling to and
fro from the server because the capabilities of the service are mapped to the
URIs and protocols. Secondly, there is no need to have a proxy at the client
end because its only data that is coming and the application can directly
receive and process the data.
WCF REST services
Windows Communication Foundation (WCF) came into
existence much later than the web service. It provided a much secure and mature
way of creating the services to achieve whatever we were not able to
achieve using traditional web services, i.e., other protocols support and even
duplex communication. With WCF, we can define our service once and then
configure it in such a way that it can be used via HTTP, TCP, IPC, and even
Message Queues. We can even configure WCF services to create REST services too
i.e. WCF REST Services.
Note: Refer
this article for details on WCF REST services: A Beginner’s Tutorial for
Understanding Windows Communication Foundation (WCF)[^]
The problem with using WCF restful services is
that we need to do a lot of configurations in a WCF service to make it a
RESTful service. WCF is suited for he scenarios where we want to create a
services that should support special scenarios such as one way messaging,
message queues, duplex communication or the services that need to conform to
WS* specifications.
But using WCF for creating restful services that
will provide fully resource oriented services over HTTP is a little complex.
Still WCF is the only option for creating the RESTful services if there is a
limitation of using .NET 3.5 framework.
Introducing ASP.NET Web API
ASP.NET
MVC - Web API. ASP.NET Web API is a framework that makes it easy to build HTTP
services that reach a broad range of clients, including browsers and mobile
devices. ASP.NET Web API is an ideal platform for building RESTful applications
on the .NET Framework. it is used to develop HTTP based web services on the top
of .NET Framework.
Microsoft
came up with ASP.NET Web API quite recently to facilitate the creation of
RESTful services that are capable of providing fully resource oriented services
for a broad range of clients including browsers, mobiles and tablets.
ASP.NET Web API is a framework for building REST services easily and in a
rather simple way.
Today, a web-based application is not enough to reach all its customers or
users. Now a day, Peoples become very smart that means they are using iPhone,
mobile, tablets, etc. devices in their daily life. These devices are having a
lot of useful apps for making their life easy. Actually, in the simple word we
can say that we are moving from the web towards the apps world. So, if we like
to expose our service data to the browsers as well as to all these modern
devices apps in a fast, secure and simple way, we should have an API which is
compatible with all browsers as well as all these modern days devices. ASP.Net
Web API is a framework to build HTTP services which can be consumed by cross
platform clients including desktops or mobile devices irrespective of the
Browsers or Operating Systems being used. ASP.Net Web API supports RESTful
applications and uses GET, PUT, POST, DELETE verbs for client communications.
What is Web API? ASP.NET Web API is a powerful platform
for building HTTP enabled service APIs that expose service and data. It can be
consumed by a broad range of clients including browsers, mobiles, desktop and
tablets. As it is HTTP service, so it can reach a broad range of client.
ASP.NET Web API is very much similar to ASP.NET MVC because it contains the
ASP.NET MVC feature like routing, controllers, action results, filter, model,
etc. Note ASP.NET Web API is not a part of MVC framework. It is a part of the
core ASP.NET. You can use Web API with ASP.NET MVC or any other type of web
application. You can also create a stand-alone service using the Web API. Note:
Using ASP.NET Web API we can only create HTTP services which are non-SOAP
based.
Why
do we need Web API?
The ASP.NET Web API is a great
framework provided by Microsoft for building HTTP services that can be consumed
by a broad range of clients including browsers, mobiles, iPhone, and tablets,
etc. Moreover, ASP.NET Web API is open source and
an ideal platform for building REST-full services over the .NET Framework.
These sets of articles are suitable for beginners
as well as experienced ASP.NET Web API developers who want to
learn ASP.NET Web API from scratch with real-time examples.
The
articles start from the very basics of ASP.NET Web API and
cover advanced concepts as we progress. If you are confident with the
concepts that are going to discuss here, I am sure, you will be in a much
better position to answer most of the interview questions as well as you can
develop any real-time applications using ASP.NET Web API.
As you know, today we all are connected with internet. Only web based
application is not enough to reach to everyone. Now a days, we all are using
apps through mobile devices, tablets which makes our life easy. So, if you want
to expose your service to everyone which is accessible on browser as well as
modern devices [Mobiles and Tablets Apps] and run fast then you need to expose
your service as API which is compatible with every browser as well as these
modern devices. ASP.NET Web API uses the full features of HTTP like
request/response headers, caching, versioning, etc. It is also a great platform
where you can create your REST-FUL services. You don’t need to define extra
configuration setting for different devices unlike WCF REST Services.
Microsoft came up with ASP.NET Web API quite recently to facilitate the creation of RESTful services that are capable of providing fully resource oriented services for a broad range of clients including browsers, mobiles and tablets. ASP.NET Web API is a framework for building REST services easily and in a rather simple way.
Today, a web-based application is not enough to reach all its customers or users. Now a day, Peoples become very smart that means they are using iPhone, mobile, tablets, etc. devices in their daily life. These devices are having a lot of useful apps for making their life easy. Actually, in the simple word we can say that we are moving from the web towards the apps world. So, if we like to expose our service data to the browsers as well as to all these modern devices apps in a fast, secure and simple way, we should have an API which is compatible with all browsers as well as all these modern days devices. ASP.Net Web API is a framework to build HTTP services which can be consumed by cross platform clients including desktops or mobile devices irrespective of the Browsers or Operating Systems being used. ASP.Net Web API supports RESTful applications and uses GET, PUT, POST, DELETE verbs for client communications.
What is Web API? ASP.NET Web API is a powerful platform for building HTTP enabled service APIs that expose service and data. It can be consumed by a broad range of clients including browsers, mobiles, desktop and tablets. As it is HTTP service, so it can reach a broad range of client. ASP.NET Web API is very much similar to ASP.NET MVC because it contains the ASP.NET MVC feature like routing, controllers, action results, filter, model, etc. Note ASP.NET Web API is not a part of MVC framework. It is a part of the core ASP.NET. You can use Web API with ASP.NET MVC or any other type of web application. You can also create a stand-alone service using the Web API. Note: Using ASP.NET Web API we can only create HTTP services which are non-SOAP based.
As you know, today we all are connected with internet. Only web based application is not enough to reach to everyone. Now a days, we all are using apps through mobile devices, tablets which makes our life easy. So, if you want to expose your service to everyone which is accessible on browser as well as modern devices [Mobiles and Tablets Apps] and run fast then you need to expose your service as API which is compatible with every browser as well as these modern devices. ASP.NET Web API uses the full features of HTTP like request/response headers, caching, versioning, etc. It is also a great platform where you can create your REST-FUL services. You don’t need to define extra configuration setting for different devices unlike WCF REST Services.
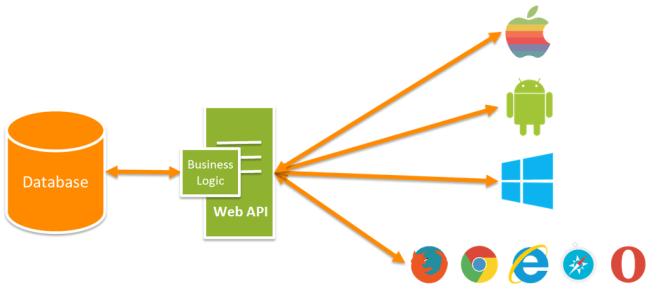
Note:
I want to clear one misconception that Web API does not replace to WCF. WCF is still a powerful programming model for creating SOAP based services which supports a variety of transport protocols like HTTP, TCP, Named Pipes or MSMQ etc.et's start
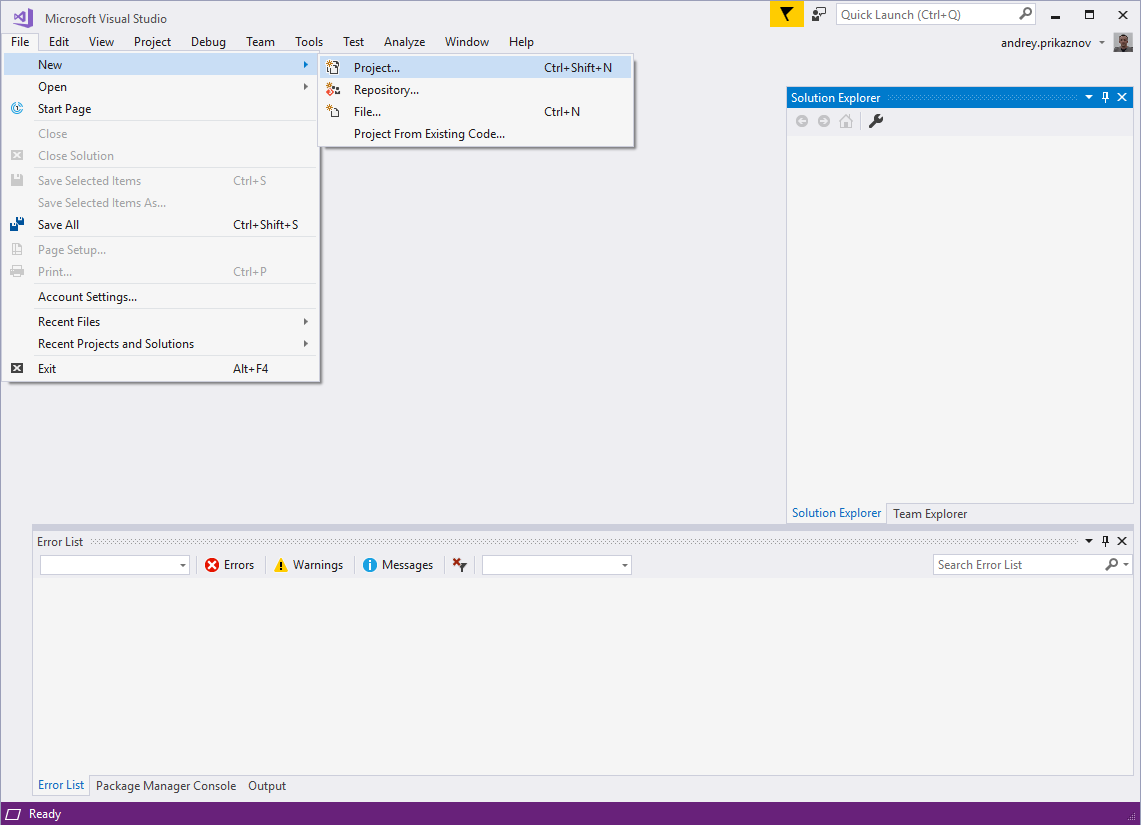


Step 1: Create a New Project
Open Microsoft Visual Studio and create a new project (File -> New -> Project). Select the "Installed" Templates, select Visual C#, then select Web. In the list of available templates, select ASP.NET Web Application (.NET Framework). Give your project a name (for my demo, I put "webapi"), then click OK.
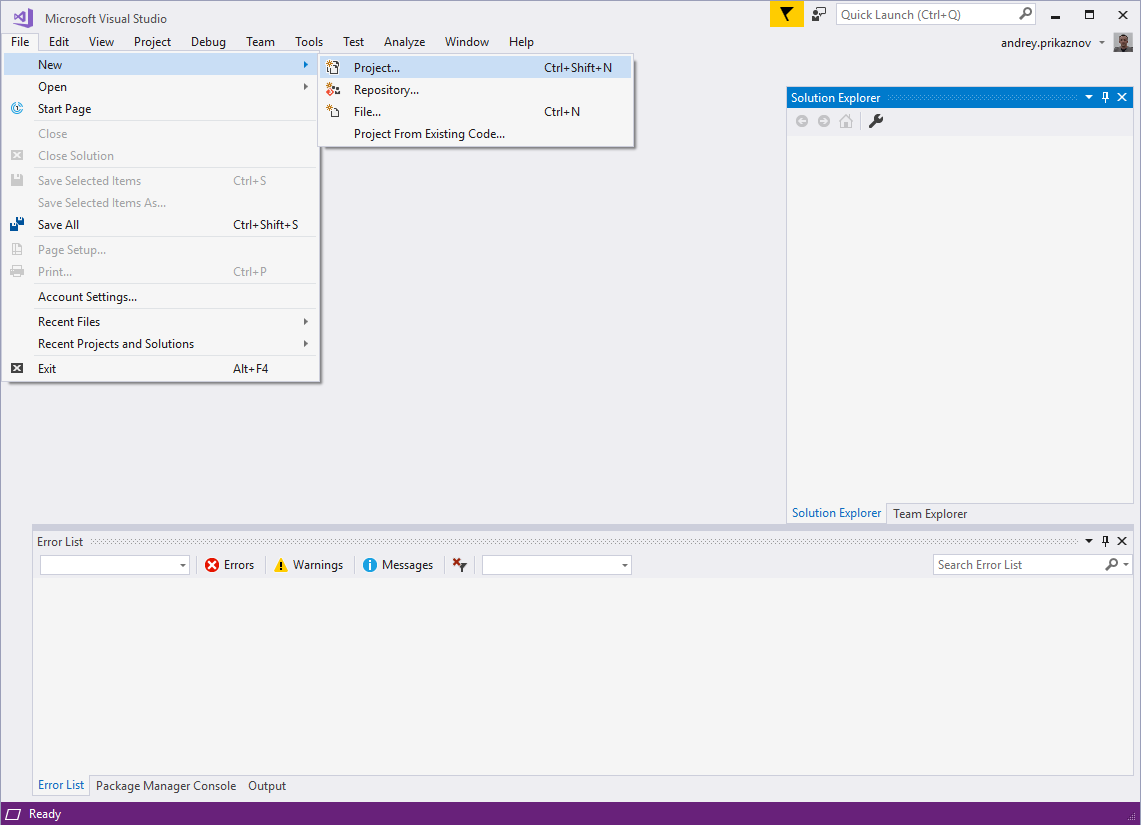
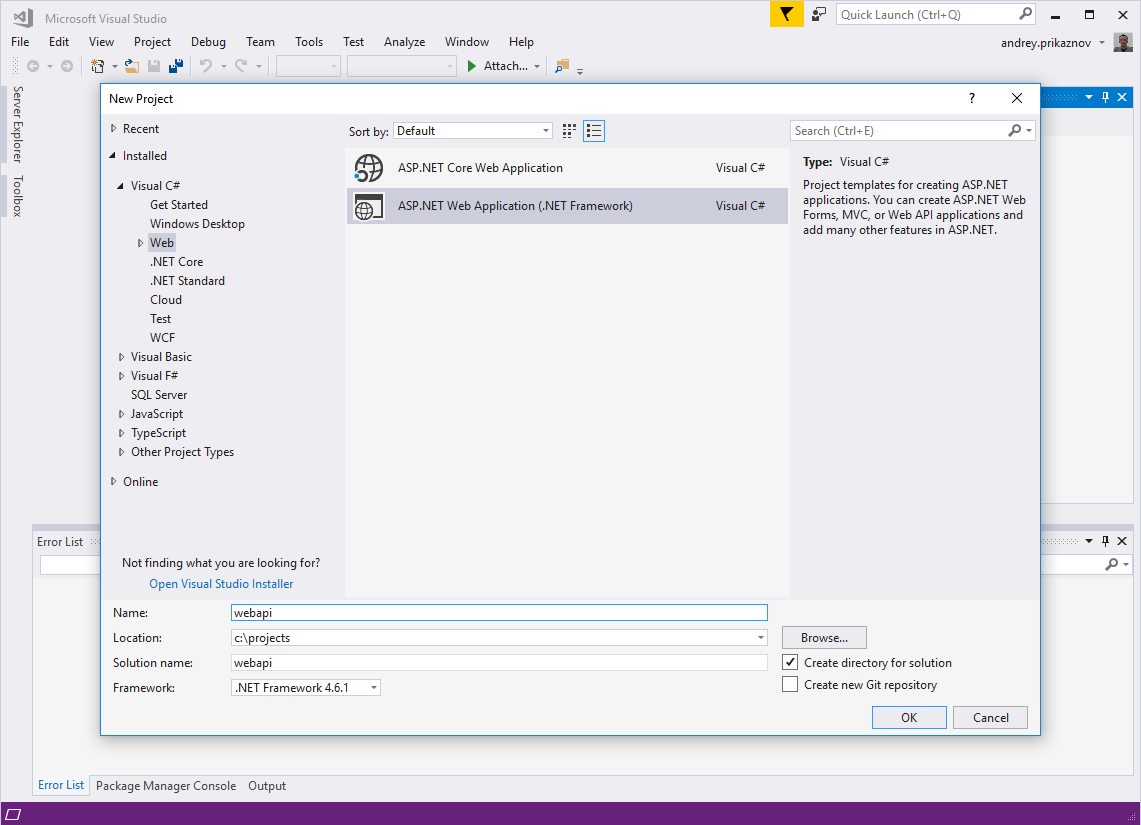
In the next modal dialog, you may choose any suitable template. Let's select Web API, so it will prepare all the basic, initial files for the project. Click OK.

Done. Now you can browse the generated folders and files in the Solution Explorer. There are application configs, help page data, a few controllers, fonts, and CSS and JS files.

Routing Table
By default, the server uses the Routing Table located in App_Start/WebApiConfig.cs.
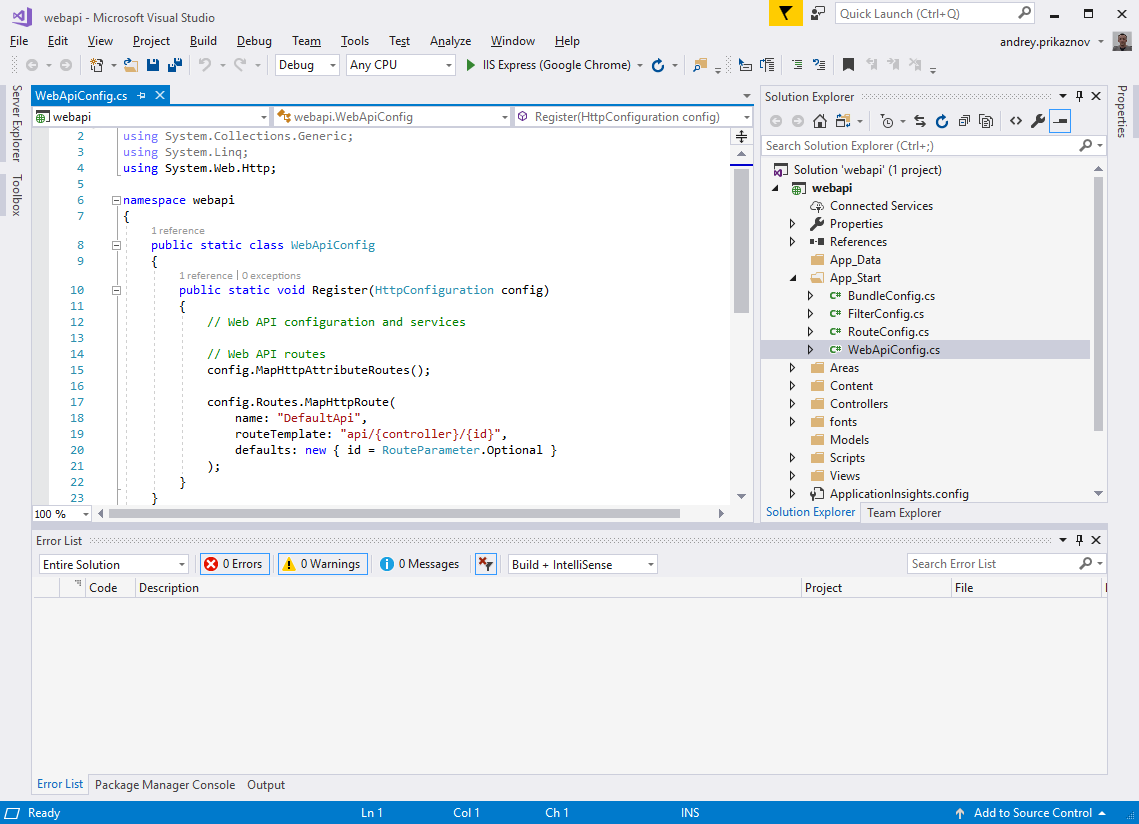
Pay attention to
routeTemplate: "api/{controller}/{id}"
, it explains the API routing.
Now, let's make a basic example. In this tutorial, we will prepare an API for users, which is a pretty general entity/object of every system.
Adding a User Model
The model represents the user, thus we will include various fields like id, name, email, phone, and role.
In Solution Explorer, right-click in the Models folder, select Add, then select Class. Then provide a class name: User. The model class is ready.


Now we just add all the fields we decided to add:
Add a User Controller
In the Web API, the controller is an object that handles all HTTP requests. In Solution Explorer, right-click the Controllers. Select Add, then select Controller.

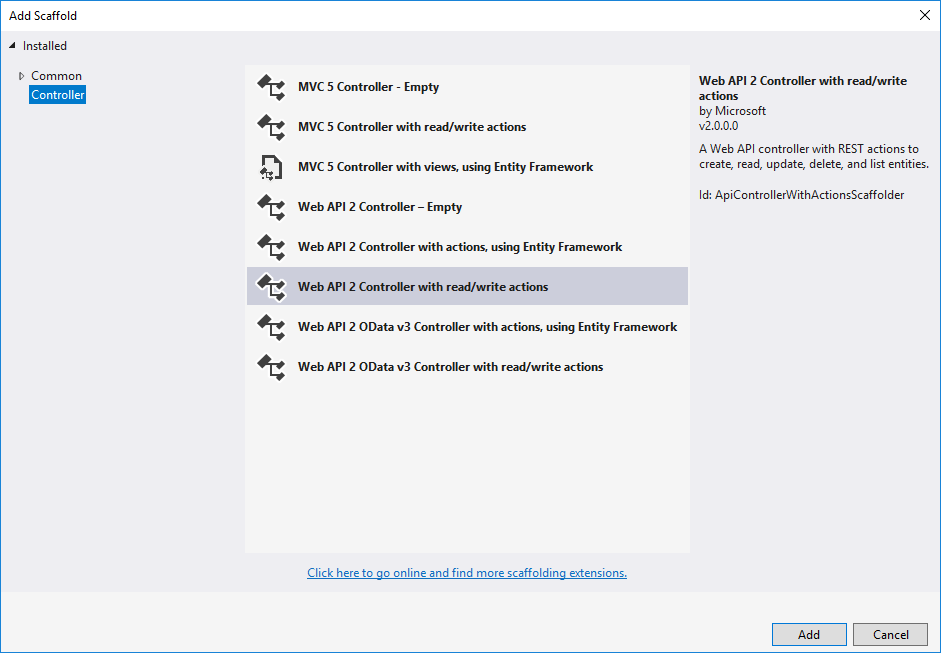
In the given dialog, select the Web API 2 Controller with read/write actions. Name the controller, UsersController. It will prepare the controller with all the proper CRUD actions.
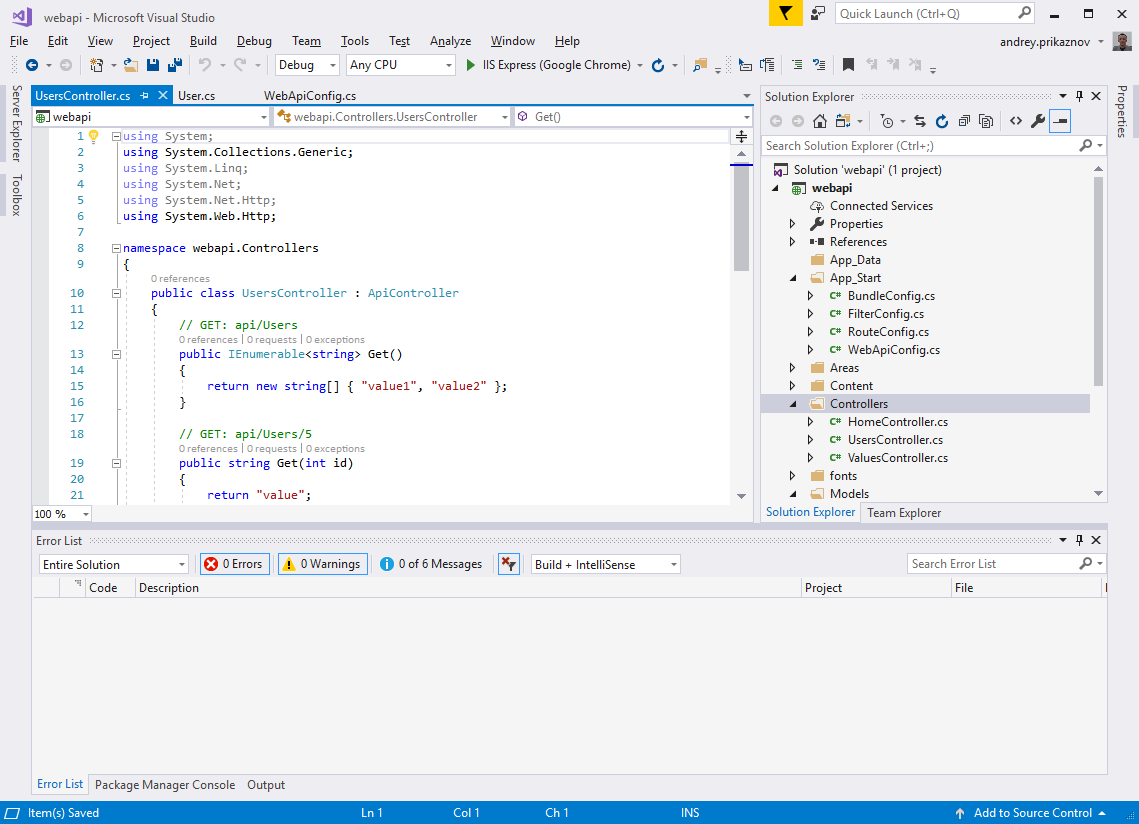
I prepared a basic example with a dummy list of users:
Step 2: Deployment
Now you can build your solution (Ctrl+Shift+B in Visual Studio). Once the build has succeeded, you can run it. Click F5 and it will open in your browser automatically at your localhost in an available port (e.g. http://localhost:61024/). Most likely, you don't want to keep it constantly running in Visual Studio, so it'd be better to keep it as service.
In this case, we can deploy it to a local dedicated server using IIS (Internet Information Services).
First, open the IIS, on the left side under Sites - add a New Website (from the right panel or right-click on the Sites). Put in the following details: Site name, "webapi.localhost.net"; Physical path, "C:\projects\webapi" (where your solution is located); Binding - http or https; the host name will be the same, i.e. "webapi.localhost.net." Click OK.
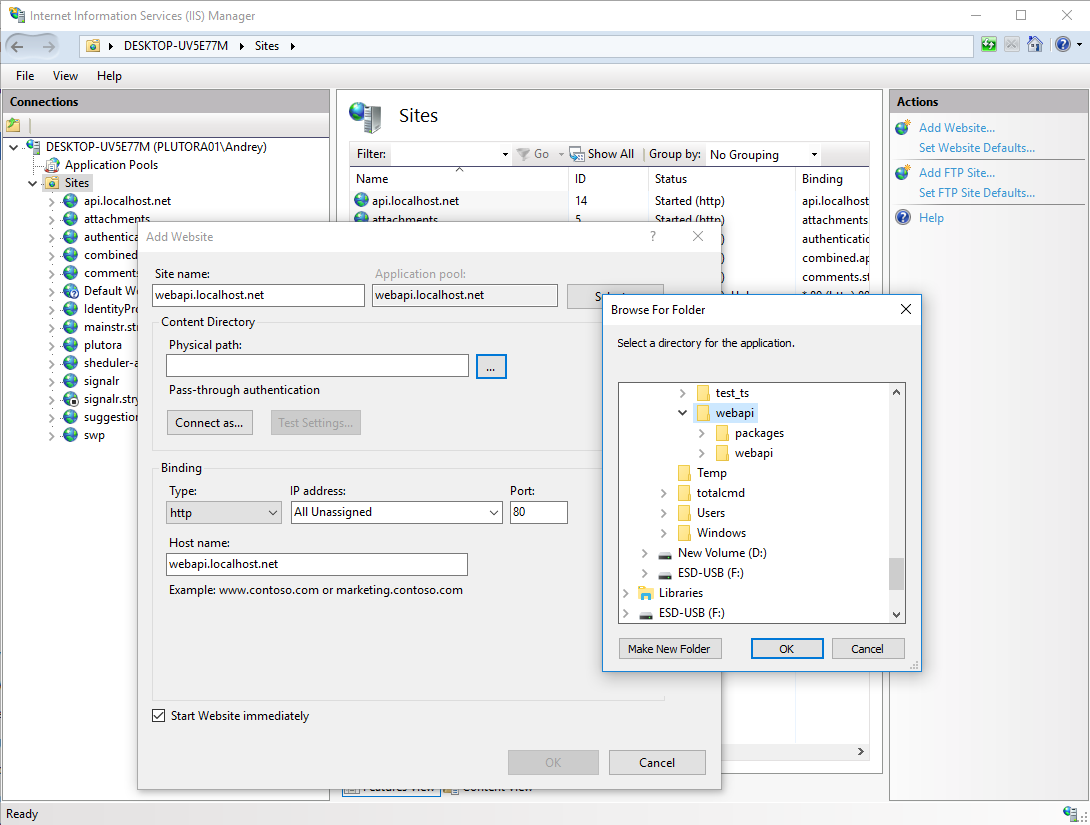
IIS should run the Web API service on webapi.localhost.net.
If you try to open webapi.localhost.net in your browser, it won't open the result we created, because the browser tries to resolve this address (webapi.localhost.net) as a global domain. In order to map this domain name with the local server, we need to modify the local hosts file. On Windows (v10) the hosts file exists in the C:\Windows\system32\drivers\etc folder. The file doesn't have its own extension, it is the "host's" file.
Copy it to another location and open it in the editor.
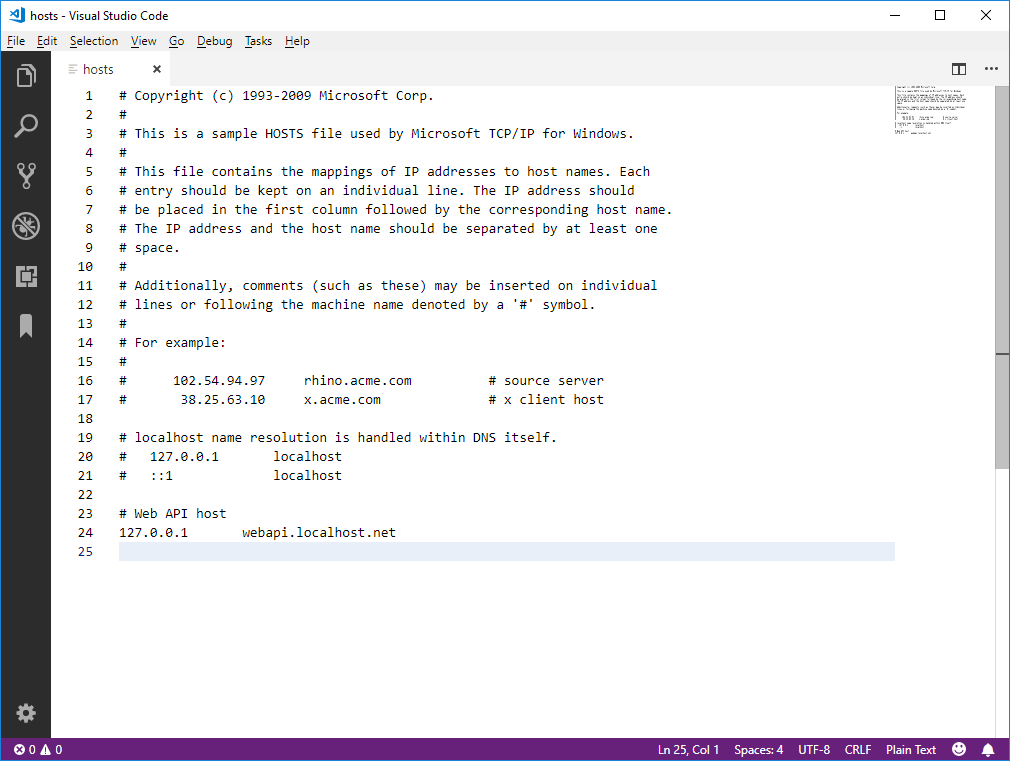
You need to add the following to the end of this file:
Now you need to put the modified file back in the C:\Windows\system32\drivers\etc folder. As this folder is protected by Windows by default, you will get an access denied warning message, so you need to copy the file "As Administrator."
After the file is updated, webapi.localhost.net should load from your localhost (C:\projects\webapi).
Testing the API
It is time to test the API methods we created for our Web server:
api/users and api/users/{id}
. Open http://webapi.localhost.net/api/users in your browser. You should get the following output: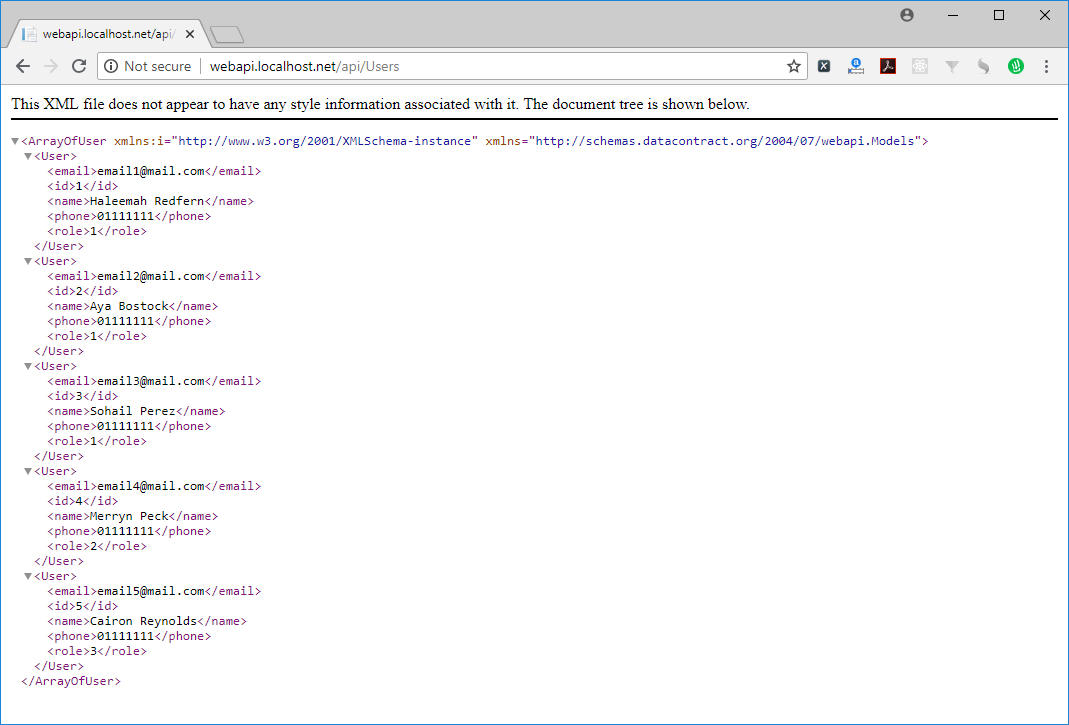
As we are creating the external API which should be accessible from outside our IDE, we need to test our API from another page. The easiest way is to do so is via the development toolbar (which exists in any modern browser). Usually it is activated when you press F12. Go to the 'Console' tab. Below I prepared two small examples you can use to test the APIs
In case jQuery is available, you can use:
Otherwise, using native JavaScript, you can use the following code:
Very likely you will receive the following error:
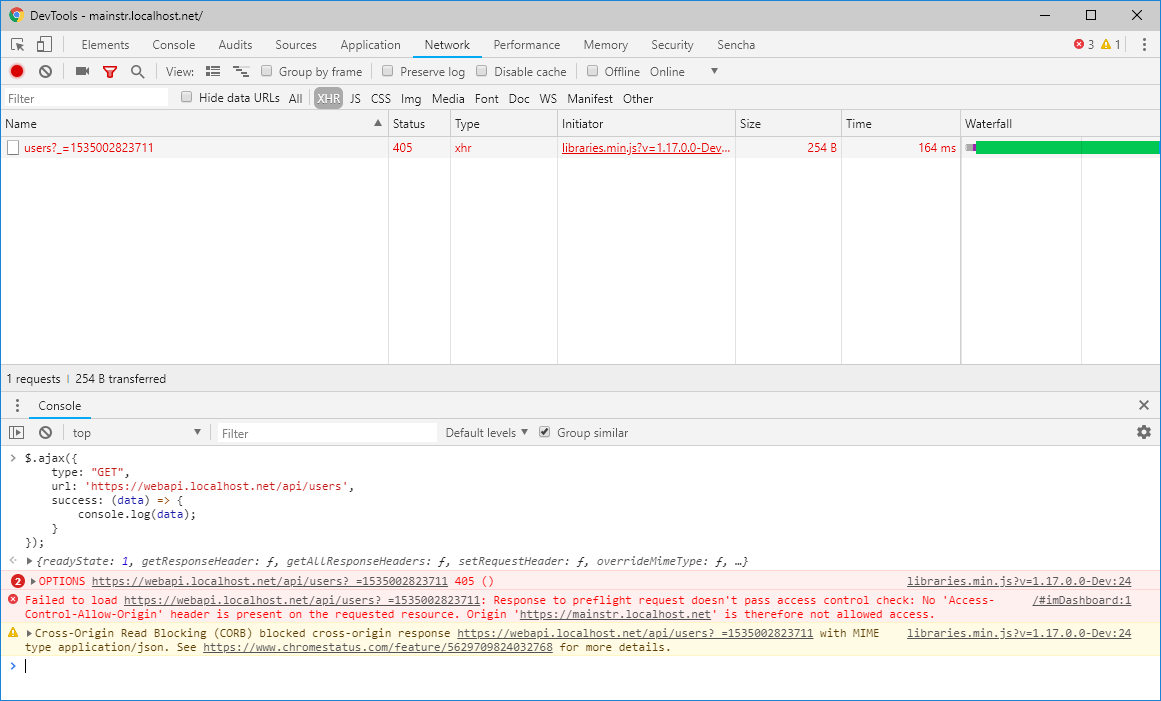
The response to the preflight request doesn't pass an access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource, because regular web pages can use the
XMLHttpRequest
object to send and receive data from remote servers, but they're limited by the same origin policy. Extensions aren't so limited. An extension can talk to remote servers outside of its origin, as long as it first requests cross-origin permissions.
Cross-Origin Resource Sharing (CORS) is a mechanism that uses additional HTTP headers to tell a browser to let a web application running at one origin (domain) have permission to access selected resources from a server at a different origin.
Adjust Cross-Origin Resource Sharing (CORS)
In order to solve this, we need to enable the CORS in our solution. In Visual Studio, open the Package Manage Console (available at the bottom of your screen, between Error List and Output). Run the following:
Install-Package Microsoft.AspNet.WebApi.Cors
This will install the WebApi.Cors reference. Then open the "App_Start\WebApiConfig.cs" file. Add
config.EnableCors();
before
config.MapHttpAttributeRoutes();

Then go back to our UsersController.cs and add
[EnableCors(origins: "*", headers: "*", methods: "*")]
before the class definition.
Finally - rebuild the project again. Then try to test the APIs again; now it should work.

No comments:
Post a Comment