What is Task in C#? ( ref: https://www.csharpstar.com/tasks-csharp/)
.NET framework provides Threading.Tasks class to let you create tasks and run them asynchronously. A task is an object that represents some work that should be done. The task can tell you if the work is completed and if the operation returns a result, the task gives you the result.
C# task object typically executes asynchronously on a thread pool thread rather than synchronously on the main application thread.

What is Thread?
.NET Framework has thread-associated classes in System.Threading namespace. A Thread is a small set of executable instructions.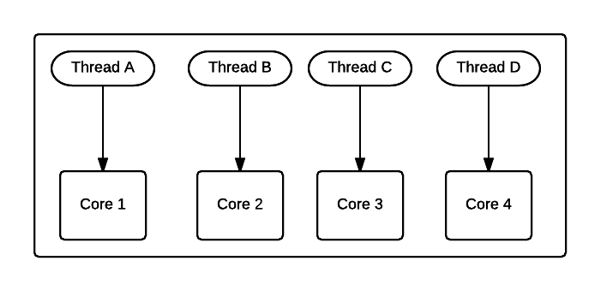

Why we need Tasks
It can be used whenever you want to execute something in parallel. Asynchronous implementation is easy in a task, using’ async’ and ‘await’ keywords.
Why we need a Thread
When the time comes when the application is required to perform few tasks at the same time.
Properties of Task Class:
Property | Description |
Exception | Returns any exceptions that caused the task to end early |
Status | Returns the Tasks status |
IsCancelled | Returns true if the Task was cancelled |
IsCompleted | Returns true if the task is completed successfully |
IsFaulted | Returns true if the task is stopped due to unhandled exception |
Factory | Provides acess to TaskFactory class. You can use that to create Tasks |
Methods in Task Class :
Methods | Purpose |
ConfigureAwait | You can use Await keyword for the Task to complete |
ContinueWith | Creates continuation tasks. |
Delay | Creates a task after specified amount of time |
Run | Creates a Task and queues it to start running |
RunSynchronously | Runs a task Synchronously |
Start | Starts a task |
Wait | Waits for the task to complete |
WaitAll | Waits until all tasks are completed |
WaitAny | Waits until any one of the tasks in a set completes |
WhenAll | Creates a Task that completes when all specified tasks are completed |
WhenAny | Creates a Task that completes when any specified tasks completes |
How to create a Task
- static void Main(string[] args) {
- Task < string > obTask = Task.Run(() => (
- return“ Hello”));
- Console.WriteLine(obTask.result);
- }
How to create a Thread
- static void Main(string[] args) {
- Thread thread = new Thread(new ThreadStart(getMyName));
- thread.Start();
- }
- public void getMyName() {}
Differences Between Task And Thread
- The Thread class is used for creating and manipulating a thread in Windows. A Task represents some asynchronous operation and is part of the Task Parallel Library, a set of APIs for running tasks asynchronously and in parallel.
- The task can return a result. There is no direct mechanism to return the result from a thread.
- Task supports cancellation through the use of cancellation tokens. But Thread doesn't.
- A task can have multiple processes happening at the same time. Threads can only have one task running at a time.
- We can easily implement Asynchronous using ’async’ and ‘await’ keywords.
- A new Thread()is not dealing with Thread pool thread, whereas Task does use thread pool thread.
- A Task is a higher level concept than Thread.
- One of the major difference between task and thread is the propagation of exception. While using thread if we get the exception in the long running method it is not possible to catch the exception in the parent function but the same can be easily caught if we are using tasks.
No comments:
Post a Comment